API Specification
API specifications are crucial. They provide standard rules and guidelines for integrating an API and serve as a single source of truth for software engineers.
In this section, you will learn:
- What is API Specification
- Why do you need an API specification and,
- Types of API Specification
What is API Specification?
An API specification is a standardized format that describes the behavior and elements of an API, ensuring clear communication and integration among developers.
The specification describes endpoints, operations on each endpoint, query and path parameters, methods, schemas, version descriptions, and more.
All these elements are compiled into a specification file, which is usually created during the development stage of the API. However, APIs with predefined specifications by governing bodies are exceptions.
API specifications are technical and often lack detailed explanations, requiring technical writers to provide comprehensive descriptions translated into API documentation. While specifications outline API elements, they don’t instruct on usage.
Note: An API specification is not the same as an API architectural style
Why do you need an API Specification?
An API specification offers clear, standardized guidelines detailing how an API should operate and interact with other software components. It ensures consistent communication by defining expected behavior, data formats, and protocols. This eliminates the need for developers to rely on internal implementation details.
Moving further, a well-defined API specification fosters collaboration among teams. It minimizes misunderstanding and reduces the time spent on API integration.
In addition, an API specification serves as a single source of truth that can be easily shared and modified, simplifying the development process. As a result, integration becomes significantly easier when APIs are clearly defined. Third-party developers can integrate their products seamlessly by referring to the specifications rather than relying on trial and error.
Finally, security is essential in API development. An API specification enables developers to implement essential security measures, such as authentication, rate limiting, and encryption, to protect sensitive data.
Types of API Specification
There are various types of API specifications with different formats
- Open API Specification(OAS)
- Restful API Modelling Language(RAML)
- API Blueprint
- GRAPHQL Schema Definition Language(SDL)
- Web Application Description Language(WADL)
This section will focus on the first three API specification types.
Open API Specification
OAS, previously known as the Swagger specification, is a format for describing, producing, consuming, and visualizing restful web services. It evolved from the Swagger framework and is now managed by the Open API Initiative(OAI) under the Linux Foundation. It is an open-source collaboration project of the Linux Foundation.
This specification defines the structure and syntax of REST APIs, making it easy for developers to build consistent APIs that adhere to Industry standards. One of the most significant advantages of open APIs is that they are language agnostic. This means that humans and computers can identify and understand service capabilities without requiring additional documentation or access to source code.
An Open API file, sometimes called an Open API document, describes an API, including endpoints, authentication methods, operation parameters, and contact information. It can be written in YAML or JSON. Big organizations like Google, Postman, Oracle, and more use the OAS.
Top-Level Structure of an Open API Specification
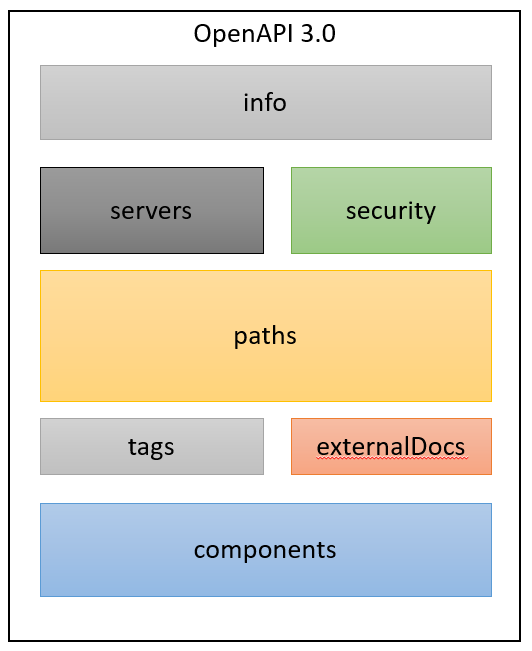
Image Source:Swagger.io
- openapi: This section specifies the version of the OAS. The OAS has different versions.
Note
You can write your OAS in YAML or JSON. I will use YAML for the example descriptions. One thing you should know about YAML is that it is indentation-sensitive.
1openapi: 3.0.0
- info: This section provides metadata about the API, including title, description, version, contact, license, etc. It gives a general overview of what the API does.
1
2info:
3 description: |
4 This is a sample Petstore server. You can find
5 out more about Swagger at
6 [http://swagger.io](http://swagger.io) or on
7 [irc.freenode.net, #swagger](http://swagger.io/irc/).
8 version: "1.0.0"
9 title: Swagger Petstore
10 termsOfService: 'http://swagger.io/terms/'
11 contact:
12 email: apiteam@swagger.io
13 license:
14 name: Apache 2.0
15 url: 'http://www.apache.org/licenses/LICENSE-2.0.html'
16
- servers: This section defines the base URLs for accessing the API. You can add multiple server URLs, such as production and testing environments, as supported by OAS 3.0
1 servers:
2 - url: 'https://petstore.swagger.io/v2'
- tags: This section contains a collection or group of related operations. Tags can also be added to path operations to add clear descriptions. For example, the pet store API has tags like user, pet, store, and more.
1tags:
2 - name: pet
3 description: Everything about your Pets
- paths: This section lists all the API paths/endpoints available and the HTTP methods supported for each path. It contains request and response objects.
1paths:
2 /pets:
3 get:
4 summary: List all pets
5 operationId: listPets
6 tags:
7 - pet
8 parameters:
9 - name: limit
10 in: query
11 description: How many items to return at one time (max 100)
12 required: false
13 schema:
14 type: integer
15 format: int32
16 responses:
17 '200':
18 description: A paged array of pets
19 content:
20 application/json:
21 schema:
22 type: array
23 items:
24 $ref: '#/components/schemas/Pet'
25 'default':
26 description: unexpected error
27 content:
28 application/json:
29 schema:
30 $ref: '#/components/schemas/Error'
31 /pets/{petId}:
32 get:
33 summary: Info for a specific pet
34 operationId: showPetById
35 tags:
36 - pet
37 parameters:
38 - name: petId
39 in: path
40 required: true
41 description: The id of the pet to retrieve
42 schema:
43 type: integer
44 format: int64
45 responses:
46 '200':
47 description: Expected response to a valid request
48 content:
49 application/json:
50 schema:
51 $ref: '#/components/schemas/Pet'
52 'default':
53 description: unexpected error
54 content:
55 application/json:
56 schema:
57 $ref: '#/components/schemas/Error'
58
- components: This section holds reusable objects for parameters, schemas, responses, etc. These components can be referenced in any path item.
1components:
2 securitySchemes:
3 petstore_auth:
4 type: oauth2
5 flows:
6 implicit:
7 authorizationUrl: 'http://petstore.swagger.io/oauth/dialog'
8 scopes:
9 write:pets: modify pets in your account
10 read:pets: read your pets
11 api_key:
12 type: apiKey
13 name: api_key
14 in: header
15 schemas:
16 Pet:
17 type: object
18 required:
19 - id
20 - name
21 properties:
22 id:
23 type: integer
24 format: int64
25 name:
26 type: string
27 tag:
28 type: string
29 Error:
30 type: object
31 required:
32 - code
33 - message
34 properties:
35 code:
36 type: integer
37 format: int32
38 message:
39 type: string
- Security: This section defines the security mechanisms that can be used across the API and includes information on authentication and authorization. The OAS supports various security mechanisms, such as 0Auth2, API Key, OpenID, HTTP for basic bearer, and other authentication schemes.
1
2security:
3 - petstore_auth: []
4 - api_key: []
5
- External Docs: This section allows you to reference external documentation by adding links.
1externalDocs:
2 description: Find out more
3 url: 'http://swagger.io'
Here is the full example code. You can preview it using the Swagger editor.
1openapi: 3.0.0
2info:
3 description: |
4 This is a sample Petstore server. You can find
5 out more about Swagger at
6 [http://swagger.io](http://swagger.io) or on
7 [irc.freenode.net, #swagger](http://swagger.io/irc/).
8 version: "1.0.0"
9 title: Swagger Petstore
10 termsOfService: 'http://swagger.io/terms/'
11 contact:
12 email: apiteam@swagger.io
13 license:
14 name: Apache 2.0
15 url: 'http://www.apache.org/licenses/LICENSE-2.0.html'
16servers:
17 - description: SwaggerHub API Auto Mocking
18 url: https://virtserver.swaggerhub.com/JudyPearls/Pet/1.0.0
19 - url: 'https://petstore.swagger.io/v2'
20tags:
21 - name: pet
22 description: Everything about your Pets
23 externalDocs:
24 description: Find out more
25 url: 'http://swagger.io'
26
27paths:
28 /pets:
29 get:
30 summary: List all pets
31 operationId: listPets
32 tags:
33 - pet
34 parameters:
35 - name: limit
36 in: query
37 description: How many items to return at one time (max 100)
38 required: false
39 schema:
40 type: integer
41 format: int32
42 responses:
43 '200':
44 description: A paged array of pets
45 content:
46 application/json:
47 schema:
48 type: array
49 items:
50 $ref: '#/components/schemas/Pet'
51 'default':
52 description: unexpected error
53 content:
54 application/json:
55 schema:
56 $ref: '#/components/schemas/Error'
57 /pets/{petId}:
58 get:
59 summary: Info for a specific pet
60 operationId: showPetById
61 tags:
62 - pet
63 parameters:
64 - name: petId
65 in: path
66 required: true
67 description: The id of the pet to retrieve
68 schema:
69 type: integer
70 format: int64
71 responses:
72 '200':
73 description: Expected response to a valid request
74 content:
75 application/json:
76 schema:
77 $ref: '#/components/schemas/Pet'
78 'default':
79 description: unexpected error
80 content:
81 application/json:
82 schema:
83 $ref: '#/components/schemas/Error'
84
85
86components:
87 securitySchemes:
88 petstore_auth:
89 type: oauth2
90 flows:
91 implicit:
92 authorizationUrl: 'http://petstore.swagger.io/oauth/dialog'
93 scopes:
94 write:pets: modify pets in your account
95 read:pets: read your pets
96 api_key:
97 type: apiKey
98 name: api_key
99 in: header
100 schemas:
101 Pet:
102 type: object
103 required:
104 - id
105 - name
106 properties:
107 id:
108 type: integer
109 format: int64
110 name:
111 type: string
112 tag:
113 type: string
114 Error:
115 type: object
116 required:
117 - code
118 - message
119 properties:
120 code:
121 type: integer
122 format: int32
123 message:
124 type: string
125security:
126 - petstore_auth: []
127 - api_key: []
Here is how the preview will look like
.png?raw=true)
0AS Tools
SwaggerHub: SwaggerHub is a collaborative platform developed by SmartBear that allows teams to design, manage, and publish APIs using the OAS. It offers a premium version of the open-source Swagger tools, providing enhanced features for API documentation.
PostMan: This tool allows users to import APIs created with Swagger and other OpenAPI specifications.
Swagger Codegen: Swagger Codegen is a tool that generates client libraries, server stubs (generated code), and API documentation from an OpenAPI Specification.
Other tools include: Open API generator, API Dog, and more.
RAML Specification
RAML, which stands for RESTful API Modeling Language, is a specification language designed to describe RESTful APIs in a structured and human-readable format. It utilizes YAML (YAML Ain’t Markup Language) as its syntax, making it accessible for developers and non-developers to understand and use.
The language’s focus on modeling rather than documenting APIs has set it apart from other specifications like OAS. This distinction has led many organizations to adopt RAML for their API design processes, particularly during the early stages of development.
With the rise in adoption of the OAS, RAML is no longer popular.
Brief History of RAML
RAML was first introduced in 2013. Its goal was to provide a more effective way to design APIs compared to existing standards like Swagger (now OpenAPI). It was developed to facilitate API design rather than document existing APIs.
Moving on, in 2015, the Open API Initiative was established under the Linux Foundation to standardize HTTP API descriptions. While RAML was considered, Swagger became the primary focus of this initiative.
A year later, on May 16, 2016, the latest major release of RAML, version 1.0, was launched. This version introduced several enhancements aimed at improving usability and modularity.
Basic Structure of a RAML Specification
A basic RAML specification starts with a version declaration, followed by metadata about the API, and then the definition of resources and their associated methods. Here’s a breakdown of the specification structure.
- Version Declaration: In RAML, you must define the version of the document.
1#%RAML 1.0
- Metadata: This section contains detailed information about the API, such as the title, version, and base URL.
1title: e-BookMobile API
2 baseUri: http://api.e-bookmobile.com/{version}
3 version: v1
- Resource Definition: Resources represent the various API endpoints. Each resource is defined with a leading slash and can have nested resources.
1 /users:
2 /authors:
3 /books:
- Method: Each resource supports various HTTP methods (GET, POST, PUT, DELETE), which are defined under their respective resources.
1 /books:
2 get:
- Query Parameter: In RAML, you can define query parameters for a resource or method to filter, sort, or paginate the returned data.
1 /books:
2 get:
3 queryParameters:
4 author:
5 displayName: Author
6 type: string
7 description: An author's full name
8 example: Mary Roach
9 required: false
10 publicationYear:
11 displayName: Pub Year
12 type: number
13 description: The year released for the first time in the US
14 example: 1984
15 required: false
16 rating:
17 displayName: Rating
18 type: number
19 description: Average rating (1-5) submitted by users
20 example: 3.14
21 required: false
22 isbn:
23 displayName: ISBN
24 type: string
25 minLength: 10
26 example: 0321736079
27 required: false
- Responses: Responses define the expected outcomes of API calls, including HTTP status codes and response bodies.
1 /{bookTitle}:
2 get:
3 description: Retrieve a specific book title
4 responses:
5 200:
6 body:
7 application/json:
8 example: |
9 {
10 "data": {
11 "id": "SbBGk",
12 "title": "Stiff: The Curious Lives of Human Cadavers",
13 "description": null,
14 "datetime": 1341533193,
15 "genre": "science",
16 "author": "Mary Roach",
17 "link": "http://e-bookmobile.com/books/Stiff"
18 },
19 "success": true,
20 "status": 200
21 }
Here is the full code example
1 #%RAML 1.0
2 ---
3 title: e-BookMobile API
4 baseUri: http://api.e-bookmobile.com/{version}
5 version: v1
6 /users:
7 /authors:
8 /books:
9 get:
10 queryParameters:
11 author:
12 displayName: Author
13 type: string
14 description: An author's full name
15 example: Mary Roach
16 required: false
17 publicationYear:
18 displayName: Pub Year
19 type: number
20 description: The year released for the first time in the US
21 example: 1984
22 required: false
23 rating:
24 displayName: Rating
25 type: number
26 description: Average rating (1-5) submitted by users
27 example: 3.14
28 required: false
29 isbn:
30 displayName: ISBN
31 type: string
32 minLength: 10
33 example: 0321736079
34 required: false
35 /{bookTitle}:
36 get:
37 description: Retrieve a specific book title
38 responses:
39 200:
40 body:
41 application/json:
42 example: |
43 {
44 "data": {
45 "id": "SbBGk",
46 "title": "Stiff: The Curious Lives of Human Cadavers",
47 "description": null,
48 "datetime": 1341533193,
49 "genre": "science",
50 "author": "Mary Roach",
51 "link": "http://e-bookmobile.com/books/Stiff"
52 },
53 "success": true,
54 "status": 200
55 }
56
Preview Documentation with RAML Github Play Ground
.png?raw=true)
RAML Tools
API Designer: A web-based editor for creating and sharing RAML API specifications. It provides suggestions for elements and highlights errors in the RAML document.
RAML TO OAS*: This tool converts RAML API specifications to OpenAPI specifications.
Other tools include RAML parser, raml2html, API Console, and more. Visit the RAML Project page to learn more about RAML tools.
API Blueprint Specification
API Blueprint is a high-level API specification language designed for creating and documenting web APIs. It uses a syntax similar to Markdown, known as MSON (Markdown Syntax for Object Notation), which makes it easy for humans to read and write while still being processable by machines.
API Blueprint is ideal for projects that need clear documentation and rapid prototyping. The file is saved with a .apib extension.
Basic Structure of an API Blueprint Specification
- Metadata: This section includes general information about the API, such as its title, version, and other basic information.
1 FORMAT: 1A
2 HOST: https://polls.apiblueprint.org/
- API Name & Overview Section: This section briefly describes the API, including its name and purpose.
1
2 # Poll API
3 Polls is a simple API allowing consumers to view polls and vote in them.
4
- Resource Group Section: These sections group related resources together.
1
2 ## Questions Collection [/questions]
3
- Action Sections: Define the actions that can be performed on resources, such as HTTP methods (GET, POST, etc.). Each action section can specify requests, responses, headers, and more.
1 ### List All Questions [GET]
2
3 + Response 200 (application/json)
4
5 [
6 {
7 "question": "Favourite programming language?",
8 "published_at": "2015-08-05T08:40:51.620Z",
9 "choices": [
10 {
11 "choice": "Swift",
12 "votes": 2048
13 }, {
14 "choice": "Python",
15 "votes": 1024
16 }, {
17 "choice": "Objective-C",
18 "votes": 512
19 }, {
20 "choice": "Ruby",
21 "votes": 256
22 }
23 ]
24 }
25 ]
26
27
28
29 ### Create a New Question [POST]
30
31 You may create your own question using this action. It takes a JSON
32 object containing a question and a collection of answers in the
33 form of choices.
34
35 + Request (application/json)
36
37 {
38 "question": "Favourite programming language?",
39 "choices": [
40 "Swift",
41 "Python",
42 "Objective-C",
43 "Ruby"
44 ]
45 }
46
47 + Response 201 (application/json)
48
49 + Headers
50
51 Location: /questions/2
52
53 + Body
54
55 {
56 "question": "Favourite programming language?",
57 "published_at": "2015-08-05T08:40:51.620Z",
58 "choices": [
59 {
60 "choice": "Swift",
61 "votes": 0
62 }, {
63 "choice": "Python",
64 "votes": 0
65 }, {
66 "choice": "Objective-C",
67 "votes": 0
68 }, {
69 "choice": "Ruby",
70 "votes": 0
71 }
72 ]
73 }
74
Here is the full example code
1 FORMAT: 1A
2 HOST: https://polls.apiblueprint.org/
3
4 # Poll
5
6 Polls is a simple API allowing consumers to view polls and vote in them.
7
8 ## Questions Collection [/questions]
9
10 ### List All Questions [GET]
11
12 + Response 200 (application/json)
13
14 [
15 {
16 "question": "Favourite programming language?",
17 "published_at": "2015-08-05T08:40:51.620Z",
18 "choices": [
19 {
20 "choice": "Swift",
21 "votes": 2048
22 }, {
23 "choice": "Python",
24 "votes": 1024
25 }, {
26 "choice": "Objective-C",
27 "votes": 512
28 }, {
29 "choice": "Ruby",
30 "votes": 256
31 }
32 ]
33 }
34 ]
35
36 ### Create a New Question [POST]
37
38 You may create your own question using this action. It takes a JSON
39 object containing a question and a collection of answers in the
40 form of choices.
41
42 + Request (application/json)
43
44 {
45 "question": "Favourite programming language?",
46 "choices": [
47 "Swift",
48 "Python",
49 "Objective-C",
50 "Ruby"
51 ]
52 }
53
54 + Response 201 (application/json)
55
56 + Headers
57
58 Location: /questions/2
59
60 + Body
61
62 {
63 "question": "Favourite programming language?",
64 "published_at": "2015-08-05T08:40:51.620Z",
65 "choices": [
66 {
67 "choice": "Swift",
68 "votes": 0
69 }, {
70 "choice": "Python",
71 "votes": 0
72 }, {
73 "choice": "Objective-C",
74 "votes": 0
75 }, {
76 "choice": "Ruby",
77 "votes": 0
78 }
79 ]
80 }
81
Preview Documentation on Apiary
.png?raw=true)
Tools for API Blueprint
Apiary: Provides interactive documentation, API mocking, testing suites, and collaboration features.
Dredd: This tool Validates API implementations against the API Blueprint specification. It can generate test cases based on the API Blueprint.
Drakov: A mock server that implements API Blueprint specifications. It is useful for testing and development.
To learn more about API Blueprint tools, visit API Blueprint tools.
Assignment
Use a Swagger editor (like SwaggerHub, Editor.Swagger.io) to create an OpenAPI Specification (OAS) document for the TDMB API. The document should include the following elements:
- Info: Basic information about the API (title, description, version, contact, license).
- Paths: Define the API endpoints and their supported HTTP methods (GET, POST, PUT, DELETE).
- Parameters: Describe query parameters, path parameters, and request bodies for each operation.
- Responses: Define the expected responses for each operation, including status codes and response schemas.
- Data Models: Create schemas to represent the data structures used by the API.
- Security: If authentication is required, specify the security scheme (e.g., API Key, OAuth2) and how it is used.
Documentation Preview and Generation: Use Swagger tools to preview the generated API documentation. This will give you an interactive view of the API, similar to what developers will see when using it.
Write a reflection on the experience of documenting the TDMB API using Swagger. Include the following:
- Challenges you faced.
- Key lessons learned about API documentation and Swagger.
Lastly, after review, tag @Technicalwrit6 on Twitter and LinkedIn, stating that this is your API Specification assignment.